Open command prompt in windows
then in command prompt type d:
and press enter .
and use following commands
To create folder myap -execute the following command:
D:\> mkdir myap
To go myap folder path -execute the following command:
D:\>cd myap
To create a virtual environment for your project, open a new command prompt, navigate to the myap folder where you want to create your project and then execute the following command:-
D:\myap>python -m venv env
In the command prompt, ensure your virtual environment is active, and execute the following command:
D:\myap>env\Scripts\activate
execute the following command to install django.
D:myap>pip install django
run the following command - This will create a myproject
directory in your current directory
D:\myap>django-admin startproject myproject
then go to myproject folder path type following command :-
D:\myap>cd myproejct
To create your app, make sure you’re in the your project directory & type this command:
D:\myap\myproejct>python manage.py startapp hello
That’ll create a directory hello app folder inside my_project folder:
after it open your myap folder inside it you will see my_project folder
and inside my_project folder you will see following structure:-
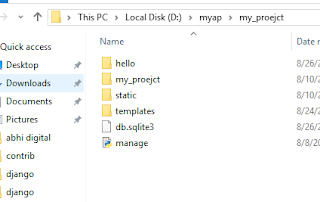
1)models.py file code:-
from django.db import models
# Create your models here.
class ContactForm(models.Model):
fullname= models.CharField(max_length=100)
email= models.EmailField()
contact= models.CharField(max_length=50)
message= models.CharField(max_length=200)
2)admin.py file code:-
from django.contrib import admin
from .models import ContactForm
# Register your models here.
admin.site.register(ContactForm)
list_display = ['fullname', 'email','contact','message' ]
3)forms.py file code:-
from django import forms
from .models import ContactForm
class FormContactForm(forms.ModelForm):
class Meta:
model= ContactForm
fields= ["fullname", "email", "contact", "message"]
widgets={'fullname':forms.TextInput(
attrs={'class':'form-control','id':'fullname'}),
'email':forms.TextInput(
attrs={'class':'form-control','id':'email'}),
'contact':forms.TextInput(
attrs={'class':'form-control','id':'contact'}),
'message':forms.Textarea(
attrs={'class':'form-control','id':'message'})
}
4)views.py file code:-
from urllib import request
from django.shortcuts import render,redirect
from hello.models import ContactForm
from hello.forms import FormContactForm
def showform(request):
form= FormContactForm(request.POST or None)
if form.is_valid():
form.save()
context= {'form': form }
return render(request, 'contactform.html', context)
or you can also write above views function code :-
def showform(request):
form= FormContactForm(request.POST or None)
if form.is_valid():
fn=request.POST['fullname']
em=request.POST['email']
cn=request.POST['contact']
msg=request.POST['message']
data=ContactForm(fullname=fn,email=em,contact=cn,message=msg)
data.save()
context= {'form': form }
return render(request, 'contactform.html', context)
5)urls.py file code (app folder ):-
from django.urls import path
from .import views
urlpatterns=[
path('',views.index,name='index'),
path('showform/', views.showform),
]
urls.py file code of Project folder (myproject):-
from django.contrib import admin
from django.urls import path, include
urlpatterns=[
path('hello/',include('hello.urls')),
path('admin/', admin.site.urls),
]
6)contactform.html file code (create this file under templates folder of your app folder ):-
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Django Contact Form</title>
<link rel="stylesheet"
href="https://maxcdn.bootstrapcdn.com/bootstrap/3.4.1/css/bootstrap.min.css">
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.4.1/jquery.min.js">
</script>
<script src="https://maxcdn.bootstrapcdn.com/bootstrap/3.4.1/js/bootstrap.min.js">
</script>
</head>
<body>
<div class="container">
<form method="POST" class="post-form" action="#">
{% csrf_token %}
{{ form.as_p }}
<div class="form-group">
<button type="submit" class="btn btn-default">Submit</button>
</div>
</div>
</form>
</body>
</html>
Sign up here with your email
ConversionConversion EmoticonEmoticon